Stable Diffusionで有名なStability AI社がプログラミング(コーディング)特化型の大規模言語モデル(LLM)の”StabilityCode”リリースしました.
StableCode
StableCodeはStability AI社が開発した大規模言語モデルです.その特徴は,プログラミング特化型のLLMであり開発者のコーディング効率を向上させるためのツールになります.
2023/08/11現在は3つのモデルが利用可能です.
- Base model:stabilityai/stablecode-completion-alpha-3b-4k
- Instruction model:stabilityai/stablecode-instruct-alpha-3b
- Long-context window model:stabilityai/stablecode-completion-alpha-3b
Base modelは,まずBigCodeのstack-dataset (v1.2) から得られた多様なプログラム言語に基づいて学習が行い,その後,Python,Go,Java,Javascript,C,markdown,C++ などの人気のある言語で追加で訓練されたモデルです.
Instruction modelは,Base modelに対して指示タスクを実現するために,Alpaca形式の約120,000のコードの指示/応答ペアで訓練したモデルです.
Long-context window modelは,ユーザーに単一または複数行の自動補完提案を提供するための最適なアシスタントとなっています.このモデルは,16,000トークンという一度に大量のコードを処理するように設計されておりユーザーが同時に最大5つの平均サイズのPythonファイルの相当分をレビューや編集することができます.
実行してみる
ここでは,StableCodeのInstruction modelをローカル環境で実行してます.
モデルのダウンロード
まずは,規約等に同意しアクセス権を得ます.
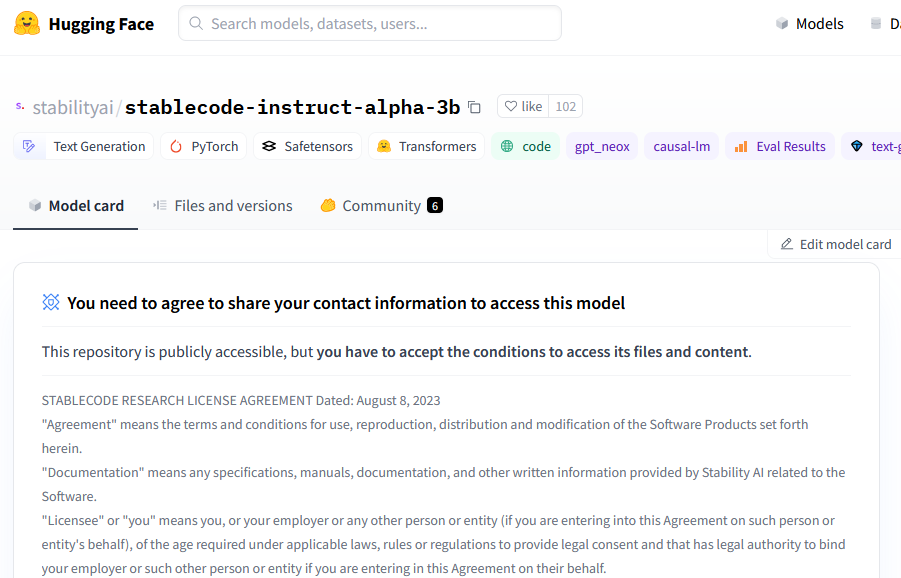

アクセス権を得た後に適当なディレクトリに移動し,モデル等をダウンロードします.
git lfs install
git clone https://huggingface.co/stabilityai/stablecode-instruct-alpha-3b
ダウンロードが完了すると次のようなディレクトリ構造となります.
stablecode-instruct-alpha-3b/
├── config.json
├── generation_config.json
├── LICENSE.md
├── model.safetensors
├── NOTICE.md
├── pytorch_model.bin
├── README.md
├── special_tokens_map.json
├── tokenizer_config.json
├── tokenizer.json
├── trainer_state.json
└── training_args.bin
これでダウンロード完了したので,実際にコードを実行してみます.
依存ライブラリのインストール
PyTorchとTransformersをインストールします.
pip install torch torchvision torchaudio transformers[torch]
サンプルコードの実行
Hugging faceのサンプルをもとに少し編集したコードを実行します.
main.py
from transformers import AutoModelForCausalLM, AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("stablecode-instruct-alpha-3b")
model = AutoModelForCausalLM.from_pretrained(
"stablecode-instruct-alpha-3b",
torch_dtype="auto",
)
model.cuda()
inputs = tokenizer(
"###Instruction\nGenerate a python function to find number of CPU cores###Response\n",
return_tensors="pt",
return_token_type_ids=False,
).to("cuda")
tokens = model.generate(
**inputs,
max_new_tokens=48,
temperature=0.2,
do_sample=True,
)
print(tokenizer.decode(tokens[0], skip_special_tokens=True))
Hugging Faceのサンプルをローカル環境で実行すると次のようなエラーが発生します.
ValueError: The following `model_kwargs` are not used by the model: ['token_type_ids'] (note: typos in the generate arguments will also show up in this list)
tokenizerにreturn_token_type_ids=False
を加えることで解消します.
結果の確認
指示したプロンプトは”Generate a python function to find number of CPU cores”(CPUコア数を取得するPython関数を書け)です.
その結果として次のような出力が得られました.
python main.py
...
###Instruction
Generate a python function to find number of CPU cores###Response
def get_cpu_count():
"""
This function will return the number of CPU cores
"""
import multiprocessing
return multiprocessing.cpu_count()
応答部のみ取り出すと次のような結果です.
def get_cpu_count():
"""
This function will return the number of CPU cores
"""
import multiprocessing
return multiprocessing.cpu_count()
正しい関数を得ることができました.
様々な例
サンプルコードだけでは面白くないため,いくつかの例を実行してみました.
C++でCPUのコア数を取得
命令:Generate a C++ function to find number of CPU cores
出力:
int getNumCores()
{
// Linux
std::ifstream cpuinfo("/proc/cpuinfo");
int numCores = 0;
std::string line;
while (std::getline(cpuinfo, line))
{
if (line.find("processor") != std::string::npos)
{
numCores++;
}
}
return numCores;
}
Linux用のコードとなってしまいましたが,要求通りのコードを生成してくれました.ただ必要なインクルードは出力してくれませんでした.
C++でCPUのコア数を取得 その2
命令:Generate a C++ program to find number of CPU cores
出力:
1. Declare an integer variable to store the number of CPU cores
2. Use the sysconf() function to get the number of CPU cores
3. Store the value of the variable with the value returned by sysconf()
4. Return the value of the variable
function > programと変更して,コード全体を書くことを期待しましたが,コードを書いてくれませんでした.
C++でCPUのコア数を取得 その3
命令:Generate a program to find number of CPU cores in C++
出力:
1. Declare an integer variable to store the number of CPU cores
2. Use the keyword 'int' to declare a variable of type 'int'
3. Use the keyword'mp_get_num_procs' to get the number of CPU cores
4. Store the value in the variable 'n'
5. Print the value of 'n' to the console
言い回しを変えてみましたが効果なし.
トークン数なども増やしてみましたが,あまり効果が得られずといった結果となってしまいました.
PythonでCPUのコア数を取得
命令:Generate a Python program to find number of CPU cores
出力:
1. Get the number of CPU cores
2. Check if the number of CPU cores is greater than 0
3. If yes, print the number of CPU cores
4. If no, print "No CPU cores found"
Pythonでも同様に行いましたがうまくいきませんでした.
まとめ
StableCodeのInstruction modelを実際に実行してみました.関数の生成はうまくいきましたが,コード全体の生成はできませんでした.
プロンプトやパラメータを調整すればもう少し改善しそうですが,指示系に関してはChatGPTほどの期待はできないようです...
実行速度も高速であることから,あくまで補完用の生成AIというところでしょう.
とはいえ,モデルパラメータは3B,モデルサイズも6GB程度と比較的手頃なサイズなので,RTX3060などのミドルエンドGPUでも実行することができます.
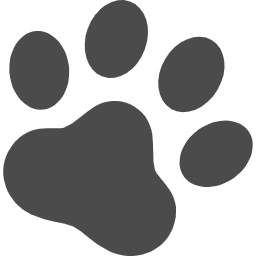
今回は取り扱いませんでしたが,コード補完系もいずれ試してみたいと思います.